WooCommerce: How to Sort Shipping Costs from Low to High
Method 1: Sorting by Total Cost
To sort shipping methods by total cost, add the following code to your functions.php file:
[php]
function wc_woocommerce_shipping_methods_by_total_cost( $rates ) {
$shipping_methods = array();
foreach ( $rates as $rate ) {
$shipping_methods[ $rate->id ] = $rate->cost + $rate->get_shipping_tax();
}
asort( $shipping_methods );
foreach ( $shipping_methods as $shipping_method_id => $shipping_method_cost ) {
foreach ( $rates as $rate ) {
if ( $rate->id == $shipping_method_id ) {
$sorted_rates[ $rate->id ] = $rate;
}
}
}
return $sorted_rates;
}
add_filter( ‘woocommerce_package_rates’, ‘wc_woocommerce_shipping_methods_by_total_cost’ );
[/php]
This code will sort shipping methods by total cost, including any taxes.
Method 2: Sorting by Base Cost
To sort shipping methods by base cost, add the following code to your functions.php file:
[php]
function wc_woocommerce_shipping_methods_by_base_cost( $rates ) {
$shipping_methods = array();
foreach ( $rates as $rate ) {
$shipping_methods[ $rate->id ] = $rate->cost;
}
asort( $shipping_methods );
foreach ( $shipping_methods as $shipping_method_id => $shipping_method_cost ) {
foreach ( $rates as $rate ) {
if ( $rate->id == $shipping_method_id ) {
$sorted_rates[ $rate->id ] = $rate;
}
}
}
return $sorted_rates;
}
add_filter( ‘woocommerce_package_rates’, ‘wc_woocommerce_shipping_methods_by_base_cost’ );
[/php]
This code will sort shipping methods by their base cost, without including any taxes.
Method 3: Sorting by Total Cost Including Free Shipping
To sort shipping methods by total cost, including free shipping, add the following code to your functions.php file:
[php]
function wc_woocommerce_shipping_methods_by_total_cost_including_free( $rates ) {
$shipping_methods = array();
foreach ( $rates as $rate ) {
$shipping_methods[ $rate->id ] = $rate->cost + $rate->get_shipping_tax();
if ( $rate->cost == 0 ) {
$shipping_methods[ $rate->id ] = 0;
}
}
asort( $shipping_methods );
foreach ( $shipping_methods as $shipping_method_id => $shipping_method_cost ) {
foreach ( $rates as $rate ) {
if ( $rate->id == $shipping_method_id ) {
$sorted_rates[ $rate->id ] = $rate;
}
}
}
return $sorted_rates;
}
add_filter( ‘woocommerce_package_rates’, ‘wc_woocommerce_shipping_methods_by_total_cost_including_free’ );
[/php]
This code will sort shipping methods by total cost, including free shipping methods with a cost of 0.
We hope these different formulas help you sort your WooCommerce shipping methods from lowest to highest cost!
Similar posts
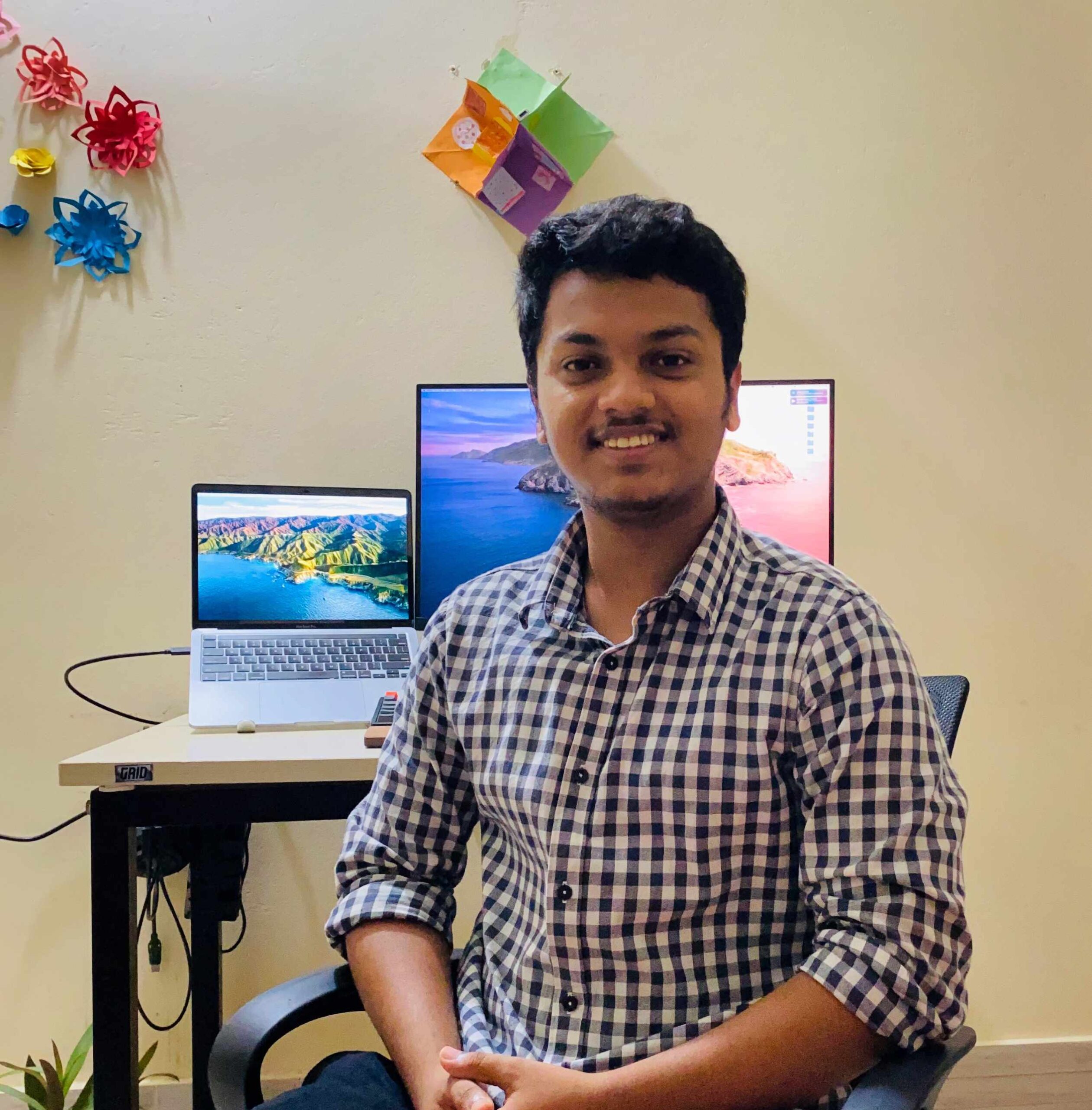
Fulltime freelance WordPress and WooCommerce Developer